dagid4
Member
I want to start a topic about concurrency in UO emulators
Hope somebody will join me. Let's start with this quotation about ServUO core usage:
According to this, ServUO is not using concurrency for world processing (player, mobiles, items). Actually, I don't know about any other community UO emulator doing it. Now several questions (feel free to add your opinion):
1. How it can be done?
I will use a quotation from Raph Koster (former lead designer of UO) answering a question: What was the technology stack driving the original Ultima Online servers?
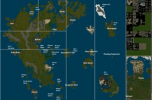
So it was done back then. But...
2. What is the main advantage?
The first advantage that comes to my mind is the ability to have more players (we're talking about thousands of players). Imagine today's 16-core processors, you could potentially divide the map into 16 boxes and have about 500 to 1000 players in each box, for a total of more than 10,000 players, assuming they don't meet in one box.
But, what shards have even more than 500 players? Maybe Outlands. This is not interesting for targeting smaller shards.
3. How else could it be useful?
Currently in ServUO, most of the BaseCreatures process (their AI) only, if there is a player near. Lets proof it:
If there is no waypoint, the default BaseCreature will check players in range:
and deactivate AI in case of no players:
within 2 sectors from the creature sector:
which is in the worst case 48 tiles.
So, it basically checks whether a player is in 32 to 48 tiles distance (depending where the creature is within the sector), otherwise it deactivates the AI.
Now, what comes to my mind. When using concurrency, we could remove this limit and process all mobiles every time, not depending on near players.
This could enable a sophisticated AI, which could simulate wolfs eating rabbits, dragon coming to village, etc. It is actually not new, Raph Koster was talking about it:
So what about using the gained CPU power via concurrency to build a sophisticated AI? What do you think, is it worth it?
Core usage does not scale linearly with demand. The server relies heavily on a single core with other jobs like save file generation and player vendor search using other cores...
According to this, ServUO is not using concurrency for world processing (player, mobiles, items). Actually, I don't know about any other community UO emulator doing it. Now several questions (feel free to add your opinion):
1. How it can be done?
I will use a quotation from Raph Koster (former lead designer of UO) answering a question: What was the technology stack driving the original Ultima Online servers?
You can see that Origin server used a multiple servers (utilizing concurrency). I have even found an image with the mentioned boxes on the map:Raph Koster said:...Each shard (the term sharding probably originated with UO) was actually multiple game servers that pointed at one persistence DB, and that did data mirroring across the boundaries. The load balancing within a shard was statically determined by config files, and was simply boxes on the map -- nothing fancy...
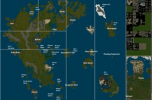
So it was done back then. But...
problems come. Supporting my opinion that it is much easier to do it single thread. Even such company as Origin had problems doing the concurrency correctly.Raph Koster said:...Race conditions here led to most of the dupe bugs, by the way...
2. What is the main advantage?
The first advantage that comes to my mind is the ability to have more players (we're talking about thousands of players). Imagine today's 16-core processors, you could potentially divide the map into 16 boxes and have about 500 to 1000 players in each box, for a total of more than 10,000 players, assuming they don't meet in one box.
But, what shards have even more than 500 players? Maybe Outlands. This is not interesting for targeting smaller shards.
3. How else could it be useful?
Currently in ServUO, most of the BaseCreatures process (their AI) only, if there is a player near. Lets proof it:
C#:
public virtual bool PlayerRangeSensitive { get { return (CurrentWayPoint == null); } }
C#:
else if (m_Owner.m_Mobile.PlayerRangeSensitive) //have to check this in the timer....
{
Sector sect = m_Owner.m_Mobile.Map.GetSector(m_Owner.m_Mobile);
if (!sect.Active)
{
m_Owner.Deactivate();
return;
}
}
C#:
private bool PlayersInRange(Sector sect, int range)
{
for (int x = sect.X - range; x <= sect.X + range; ++x)
{
for (int y = sect.Y - range; y <= sect.Y + range; ++y)
{
Sector check = GetRealSector(x, y);
if (check != m_InvalidSector && check.Players.Count > 0)
{
return true;
}
}
}
return false;
}
C#:
public const int SectorSize = 16;
public static int SectorActiveRange = 2;
So, it basically checks whether a player is in 32 to 48 tiles distance (depending where the creature is within the sector), otherwise it deactivates the AI.
Now, what comes to my mind. When using concurrency, we could remove this limit and process all mobiles every time, not depending on near players.
This could enable a sophisticated AI, which could simulate wolfs eating rabbits, dragon coming to village, etc. It is actually not new, Raph Koster was talking about it:
Raph Koster said:...
At one point, we had effects like beggars who had desires for GOLD, and thus followed rich players in the street. We had bears who would hang out in their cave, but would wander over to hang around near beehives. All that stuff worked. The problem was that the constant radial searches were incredibly expensive, and so was all the pathfinding.
One of the first things to go was the search frequency. Next, the step was taken to put every creature to “sleep” when players were not nearby. This change alone really ruins the large-scale ecological applications completely.
...
So what about using the gained CPU power via concurrency to build a sophisticated AI? What do you think, is it worth it?