Lets take sockets for example, when you spawn an item in the world the XML Socket is attached or you can attach it with a socket hammer, etc. Is there a way to hard code that XML attachment in the script so when I spawn the item in the world it is always the same?
So for example, I spawn a special shield in the game I don't want to randomly attach the socket XML as it is random, I always want to attach 5 sockets.
Hope that makes sense, let me know if you need more explanation.
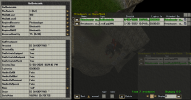
So for example, I spawn a special shield in the game I don't want to randomly attach the socket XML as it is random, I always want to attach 5 sockets.
Hope that makes sense, let me know if you need more explanation.
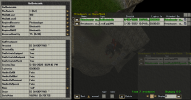