D4rkh0bb1T
Member
Hi everyone!.
My attachment name is XmlArtifactBonus.
I tweaked the attachment to give the player a bonus drop with 2 values : Bonus Multiplier and Bonus Duration.
Here, for exemple : I can AddAtt XmlArtifactBonus 2 3 which will give the targeted player a bonus drop of 2x for 3 hours. But I want more :
I want the attachment to be set by players with a gump so they buy and decide for themselves what bonus they want. Currency would be Daat's Tokens.
I created my gump with gump studio and i'm not sure where to start or how it could works for now.
Any help would be appreciated. I just began with all of this.
Here's my attachment and gump scripts :
Here's a screenshot of the gump so you can understand more what i want to achieve :
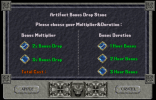
My attachment name is XmlArtifactBonus.
I tweaked the attachment to give the player a bonus drop with 2 values : Bonus Multiplier and Bonus Duration.
Here, for exemple : I can AddAtt XmlArtifactBonus 2 3 which will give the targeted player a bonus drop of 2x for 3 hours. But I want more :
I want the attachment to be set by players with a gump so they buy and decide for themselves what bonus they want. Currency would be Daat's Tokens.
I created my gump with gump studio and i'm not sure where to start or how it could works for now.
Any help would be appreciated. I just began with all of this.
Here's my attachment and gump scripts :
XmlArtifactBonus:
//By Tresdni
//www.uofreedom.com
using System;
using Server;
using Server.Items;
using Server.Network;
using Server.Mobiles;
namespace Server.Engines.XmlSpawner2
{
public class XmlArtifactBonus : XmlAttachment
{
private int m_Value = 3; // default value of 3x's drops.
[CommandProperty(AccessLevel.GameMaster)]
public int Value { get { return m_Value; } set { m_Value = value; } }
// These are the various ways in which the message attachment can be constructed.
// These can be called via the [addatt interface, via scripts, via the spawner ATTACH keyword.
// Other overloads could be defined to handle other types of arguments
// a serial constructor is REQUIRED
public XmlArtifactBonus(ASerial serial)
: base(serial)
{
}
[Attachable]
public XmlArtifactBonus()
{
}
[Attachable]
public XmlArtifactBonus(int value, double expire)
{
m_Value = value;
Expiration = TimeSpan.FromHours(expire);
}
public override void OnAttach()
{
base.OnAttach();
// apply the mod
if (AttachedTo is Mobile)
{
Mobile m = AttachedTo as Mobile;
Effects.PlaySound( m, m.Map, 516 );
m.SendMessage(String.Format("You now have {1}x artifact drops for {0} hours. Happy hunting!", Expiration.TotalHours, m_Value));
}
}
public override void OnDelete()
{
base.OnDelete();
if(AttachedTo is Mobile)
{
Mobile m = AttachedTo as Mobile;
if(!m.Deleted)
{
Effects.PlaySound( m, m.Map, 958 );
m.SendMessage(String.Format("Your increased artifact drops bonus fades away..."));
}
}
}
}
}
BonusDropStone:
using System;
using Server;
using Server.Gumps;
using Server.Engines.XmlSpawner2;
namespace Server.Gumps
{
public class BonusDropStone : Gump
{
public BonusDropStone()
: base( 0, 0 )
{
this.Closable=false;
this.Disposable=false;
this.Dragable=true;
this.Resizable=false;
this.AddPage(0);
this.AddPage(1);
this.AddBackground(191, 158, 443, 288, 9200);
this.AddBackground(198, 164, 425, 225, 9270);
this.AddImage(611, 153, 10460);
this.AddImage(611, 371, 10460);
this.AddImage(185, 371, 10460);
this.AddImage(185, 153, 10460);
this.AddImage(323, 392, 10452);
this.AddButton(520, 405, 5200, 5201, (int)Buttons.Quit, GumpButtonType.Reply, 0);
this.AddButton(220, 405, 5204, 5205, (int)Buttons.Apply, GumpButtonType.Reply, 0);
this.AddLabel(330, 184, 1149, @"Artifact Bonus Drop Stone");
this.AddLabel(245, 245, 1149, @"Bonus Multiplier");
this.AddLabel(475, 245,1149, @"Bonus Duration");
this.AddLabel(287, 213, 1149, @"Please choose your Multiplier&Duration :");
this.AddCheck(240, 273, 2151, 2154, true, (int)Buttons.2xBonus);
this.AddCheck(241, 312, 2151, 2154, false, (int)Buttons.3xBonus);
this.AddCheck(471, 273, 2151, 2154, true, (int)Buttons.1hBonus);
this.AddCheck(471, 310, 2151, 2154, false, (int)Buttons.2hBonus);
this.AddCheck(471, 348, 2151, 2154, false, (int)Buttons.3hBonus);
this.AddLabel(277, 277, 72, @"2x Bonus Drop");
this.AddLabel(277, 318, 72, @"3x Bonus Drop");
this.AddLabel(508, 277, 72, @"1 Hour Bonus");
this.AddLabel(508, 315, 72, @"2 Hour Bonus");
this.AddLabel(508, 354, 72, @"3 Hour Bonus");
this.AddLabel(245, 354, 42, @"Total Cost :");
this.AddImageTiled(413, 238, 1, 135, 9103);
}
public enum Buttons
{
Quit,
Apply,
2xBonus,
3xBonus,
1hBonus,
2hBonus,
3hBonus,
}
public override void OnResponse(NetState sender, RelayInfo info)
{
Mobile from = sender.Mobile;
switch (info.ButtonID)
{
case (int)Buttons.Quit:
{
from.SendMessage("You haven chosen to not get any bonus drop!");
from.PlaySound(0X04A);
break;
}
case (int)Buttons.Apply:
{
break;
}
case (int)Buttons.2xBonus:
{
break;
}
case (int)Buttons.3xBonus:
{
break;
}
case (int)Buttons.1hBonus:
{
break;
}
case (int)Buttons.2hBonus:
{
break;
}
case (int)Buttons.3hBonus:
{
break;
}
}
}
}
}
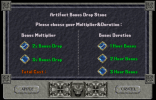