Xen
Member
Friends, Mates and Camrades, whatever,
I want to propose you a different way to generate Items, spells, Mobs without need for creating 1 script for each one of them. I'm going to propose you a good system to have Items, Spells and Mobs at runtime and edit them without writing any line of code.
1) We will use in this explication Modules -> BaseModules written by @Insanity and present in our ServUO framework. It means that this approach can be used only in ServUO (it could be a very huge difference between ServUO and RunUO)
This is a Scheme about how we will use Modules in our pattern :
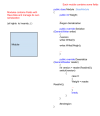
2) We will generate a Definition class for each kind of stuff we will need to generate:
I will illustrate you only one example about a knife:
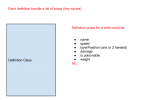
We can have a "definition" class which will include all props we need to generate all weapons we want.
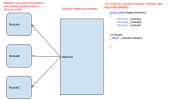
we can divide raw data in more modules or just use only one module as we like. It's not much important, it's important only what we want to do next.
3) Now we need a way to manage all definitions we want to create. I.e. we need a Core which will handle all the data we put inside it and generates items when the proper command is called.
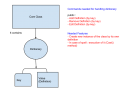
Each definition will have its own "string" keyword, so we can put it in a Dictionary. When the dictionary it's called it will grant the access to the right istance of the definition we need.
4) Class Modification or how to write the right class in the right way?
As I wrote before, each class will be rapresentative of all its istances, I.E. it will be a class to rapresent all melee weapons (or ranged weapons too it depends by you) each for all armors and so on.
And each class will have a reference to the right istance of its own definition.
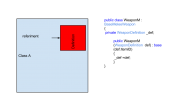
Always speaking about our knife, we will have only one class for each weapon and we just will change data inside our definitions and its own keyword.
5)
Ok let's suppose that we want to generare our Knife and we want to call it "StellarKnife"
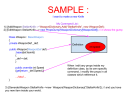
Pros :
Sample : Axe and Double Axe right now have the same identical kind of class BUT they have different values. In a normal class-oriented paradigm it could not be done.
What do you think about? Would you like to help me? Do you see what i tried to explain? Do you think it could be helpfull to ServUO projects?
Thanks for comments
I want to propose you a different way to generate Items, spells, Mobs without need for creating 1 script for each one of them. I'm going to propose you a good system to have Items, Spells and Mobs at runtime and edit them without writing any line of code.
1) We will use in this explication Modules -> BaseModules written by @Insanity and present in our ServUO framework. It means that this approach can be used only in ServUO (it could be a very huge difference between ServUO and RunUO)
This is a Scheme about how we will use Modules in our pattern :
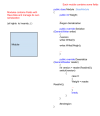
2) We will generate a Definition class for each kind of stuff we will need to generate:
- Weapons
- Armors
- Spells
- Mobs
- Talismans
- etc... etc.
I will illustrate you only one example about a knife:
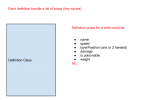
We can have a "definition" class which will include all props we need to generate all weapons we want.
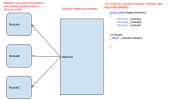
we can divide raw data in more modules or just use only one module as we like. It's not much important, it's important only what we want to do next.
3) Now we need a way to manage all definitions we want to create. I.e. we need a Core which will handle all the data we put inside it and generates items when the proper command is called.
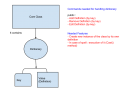
Each definition will have its own "string" keyword, so we can put it in a Dictionary. When the dictionary it's called it will grant the access to the right istance of the definition we need.
4) Class Modification or how to write the right class in the right way?
As I wrote before, each class will be rapresentative of all its istances, I.E. it will be a class to rapresent all melee weapons (or ranged weapons too it depends by you) each for all armors and so on.
And each class will have a reference to the right istance of its own definition.
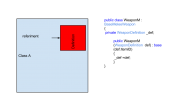
Always speaking about our knife, we will have only one class for each weapon and we just will change data inside our definitions and its own keyword.
5)
Ok let's suppose that we want to generare our Knife and we want to call it "StellarKnife"
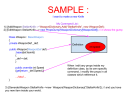
Pros :
- Items / Weapons / spells generation will be faster
- It will allow you to avoid having 20000 scripts which have only few different stuffs
Sample : Axe and Double Axe right now have the same identical kind of class BUT they have different values. In a normal class-oriented paradigm it could not be done.
Double Axe
Axe
Code:
using System;
namespace Server.Items
{
[FlipableAttribute(0xf4b, 0xf4c)]
public class DoubleAxe : BaseAxe
{
[Constructable]
public DoubleAxe()
: base(0xF4B)
{
this.Weight = 8.0;
}
public DoubleAxe(Serial serial)
: base(serial)
{
}
public override WeaponAbility PrimaryAbility
{
get
{
return WeaponAbility.DoubleStrike;
}
}
public override WeaponAbility SecondaryAbility
{
get
{
return WeaponAbility.WhirlwindAttack;
}
}
public override int AosStrengthReq
{
get
{
return 45;
}
}
public override int AosMinDamage
{
get
{
return 15;
}
}
public override int AosMaxDamage
{
get
{
return 17;
}
}
public override int AosSpeed
{
get
{
return 33;
}
}
public override float MlSpeed
{
get
{
return 3.25f;
}
}
public override int OldStrengthReq
{
get
{
return 45;
}
}
public override int OldMinDamage
{
get
{
return 5;
}
}
public override int OldMaxDamage
{
get
{
return 35;
}
}
public override int OldSpeed
{
get
{
return 37;
}
}
public override int InitMinHits
{
get
{
return 31;
}
}
public override int InitMaxHits
{
get
{
return 110;
}
}
public override void Serialize(GenericWriter writer)
{
base.Serialize(writer);
writer.Write((int)0); // version
}
public override void Deserialize(GenericReader reader)
{
base.Deserialize(reader);
int version = reader.ReadInt();
}
}
}
Axe
Code:
using System;
namespace Server.Items
{
[FlipableAttribute(0xF49, 0xF4a)]
public class Axe : BaseAxe
{
[Constructable]
public Axe()
: base(0xF49)
{
this.Weight = 4.0;
}
public Axe(Serial serial)
: base(serial)
{
}
public override WeaponAbility PrimaryAbility
{
get
{
return WeaponAbility.CrushingBlow;
}
}
public override WeaponAbility SecondaryAbility
{
get
{
return WeaponAbility.Dismount;
}
}
public override int AosStrengthReq
{
get
{
return 35;
}
}
public override int AosMinDamage
{
get
{
return 14;
}
}
public override int AosMaxDamage
{
get
{
return 16;
}
}
public override int AosSpeed
{
get
{
return 37;
}
}
public override float MlSpeed
{
get
{
return 3.00f;
}
}
public override int OldStrengthReq
{
get
{
return 35;
}
}
public override int OldMinDamage
{
get
{
return 6;
}
}
public override int OldMaxDamage
{
get
{
return 33;
}
}
public override int OldSpeed
{
get
{
return 37;
}
}
public override int InitMinHits
{
get
{
return 31;
}
}
public override int InitMaxHits
{
get
{
return 110;
}
}
public override void Serialize(GenericWriter writer)
{
base.Serialize(writer);
writer.Write((int)0); // version
}
public override void Deserialize(GenericReader reader)
{
base.Deserialize(reader);
int version = reader.ReadInt();
}
}
}
- Memory Costs, less scrips means less compilation time, less need of reflection usage and less memory usage (referecences costs only 64 bits).
- Easier to manage to not-experted people (handling a gump it's easeir than managing a script) and it can be done via client without needs of recompilation
- Serialization can be done via XML or JSon to share Items /spells /Mobs etc...
- Old style (script-based) generation for very customized items can be mantained because it doesn't need any change.
- It can be a different approach to make people understand that ServUO is not RunUO.
- It needs a gump system to make all this work well.
- It needs some way to handle (via gump) lists and collections.
- It needs some start-work to make it be worth. It needs some experience to be implemented
- It will be a very different system than RunUO and it could have some incompatibility issues with it
- Very customized script will need to be handled in the old way or it'll be needed to write down more difficult code to have different behavior for custom weapons, spells, etc...
- It will be needed some wolkaroud some Class attribute [Flippable] or [Constructable] which are written on Classes
instead in some property inside the class but it's not so impossible to handle this problem.
- Craft system should be updated (see the point before)
What do you think about? Would you like to help me? Do you see what i tried to explain? Do you think it could be helpfull to ServUO projects?
Thanks for comments
Last edited: