Tukaram
Member
Timers have never been my strong suit. But this seems odd. I am showing the code snippet with the timer, and included the whole script. I am testing the timer for the quest delay. The time delay works, but look at the pic and see what odd number she speaks. "9.49295261 more minutes"? I thought she was going to start reciting pi.
Is this normal? Is there something I can do to make the timer display different? I see it is in minutes, I thought most timers were in seconds? If I change it to 'TimeSpan.FromSeconds(1800)' would it work (I just thought of that while typing, so I will try, but post anyway).
~Edit~ Nope seconds or minutes both work for the delay, and both display the same way. Odd...
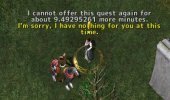
Is this normal? Is there something I can do to make the timer display different? I see it is in minutes, I thought most timers were in seconds? If I change it to 'TimeSpan.FromSeconds(1800)' would it work (I just thought of that while typing, so I will try, but post anyway).
~Edit~ Nope seconds or minutes both work for the delay, and both display the same way. Odd...
C#:
namespace Server.Engines.Quests
{
public class HavenMiners : BaseQuest
{
public override TimeSpan RestartDelay { get { return TimeSpan.FromMinutes(30); } }
public override bool DoneOnce{ get{ return false; } }
public override object Title{ get{ return "HavenMiners"; } }
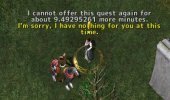
Attachments
Last edited: