Since my server is not AOS, propeties are not displayed so i had to add manually the next thing:
And this is the way it looks in game, boooo:
Thanks
Code:
//////////////////ADDED BY MED/////////////////////
public override void OnSingleClick( Mobile from )
{
if(m_Completed)
{
string CompletedText ;
bool ascii = true;
CompletedText = String.Concat("Task Completed [{0} {1}]", this.m_AmountToGather, m_MaterialName);
int hue = (1169);
PrivateOverheadMessage(MessageType.Label, hue, ascii, CompletedText /* m_AmountToGather, m_MaterialName */, from.NetState);
}
else
{
string ObtainedText ;
bool ascii = true;
ObtainedText = String.Concat( "{0} / {1} {2} Obtained", m_AmountGathered, m_AmountToGather, m_MaterialName );
int hue = (24);
PrivateOverheadMessage(MessageType.Label, hue, ascii, ObtainedText/*m_AmountGathered, m_AmountToGather , m_MaterialName*/, from.NetState);
}
}
///////////////////////////////////
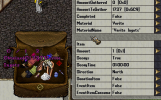
Thanks