- ServUO Version
- Publish 57
- Ultima Expansion
- Endless Journey
So I am using the XML Socketing system which works great, however is there a way to add in the scripts that when an item is created it does not get the attachment applied? I don't mind if it's socketed after creation but I don't want to spawn lets say a donation item in only to have it be socketed as this usually means it places a limit on the sockets which I do not want on certain scripted items. I hope that makes sense, let me know if I need to clarify more thank you.
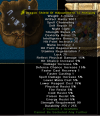
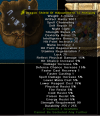